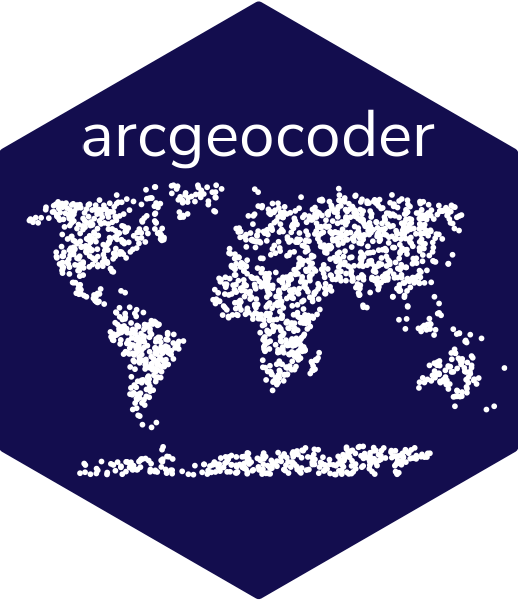
Example: arcgeocoder and leaflet maps
Combine arcgeocoder and leaflet maps
Source:vignettes/articles/ex_leaflet.Rmd
ex_leaflet.Rmd
Example
The following example shows how it is possible to create a nice leaflet map with data retrieved with arcgeocoder.
This widget is browsable and filterable thanks to crosstalk and reactable:
# Coffee Shops and Bakeries around the Eiffel Tower
library(arcgeocoder)
library(leaflet)
library(dplyr)
library(reactable)
library(crosstalk)
# Step 1: Eiffel Tower
eiffel_tower <- arc_geo_multi("Eiffel Tower",
city = "Paris", countrycode = "FR",
category = "POI"
)
# Base url for icons
icon_url <- paste0(
"https://raw.githubusercontent.com/dieghernan/arcgeocoder/",
"main/vignettes/articles/"
)
eiffel_icon <- makeIcon(
iconUrl = paste0(icon_url, "eiffel-tower.png"),
iconWidth = 50, iconHeight = 50,
iconAnchorX = 25, iconAnchorY = 25
)
# Step 2: Coffee Shops and Bakeries nearby
cf_bk <- arc_geo_categories(
category = c("Coffee Shop", "Bakery"),
x = eiffel_tower$lon, y = eiffel_tower$lat,
limit = 50,
full_results = TRUE
)
# Labels and icons
labs <- paste0("<strong>", cf_bk$PlaceName, "</strong><br>", cf_bk$StAddr)
# Assign icons
leaf_icons <- icons(
ifelse(cf_bk$Type == "Coffee Shop",
paste0(icon_url, "coffee-cup.png"),
paste0(icon_url, "croissant.png")
),
iconWidth = 20, iconHeight = 20,
iconAnchorX = 10, iconAnchorY = 10
)
# Step 3: Crosstalk object
cf_bk_data <- cf_bk %>%
select(Place = ShortLabel, Type, Address = Place_addr, City, URL, Phone) %>%
SharedData$new(group = "Food")
# Step 4: Leaflet map with crosstalk
# Init leaflet map
lmend <- leaflet(
data = cf_bk_data,
elementId = "EiffelTower", width = "100%", height = "60vh",
options = leafletOptions(minZoom = 12)
) %>%
setView(eiffel_tower$lon, eiffel_tower$lat, zoom = 16) %>%
addProviderTiles(
provider = "CartoDB.Positron",
group = "CartoDB.Positron"
) %>%
addTiles(group = "OSM") %>%
addMarkers(data = eiffel_tower, ~lon, ~lat, icon = eiffel_icon) %>%
addMarkers(
lat = cf_bk$lat, lng = cf_bk$lon, popup = labs, icon = leaf_icons
) %>%
addLayersControl(
baseGroups = c("CartoDB.Positron", "OSM"),
position = "topleft",
options = layersControlOptions(collapsed = FALSE)
)
# Step 5: Reactable for filtering
tb <- reactable(cf_bk_data,
selection = "multiple",
onClick = "select",
rowStyle = list(cursor = "pointer"),
filterable = TRUE,
searchable = TRUE,
showPageSizeOptions = TRUE,
striped = TRUE,
defaultColDef = colDef(vAlign = "center", minWidth = 150),
paginationType = "jump",
elementId = "coffees",
columns = list(
Place = colDef(
sticky = "left", rowHeader = TRUE, name = "",
cell = function(value) {
htmltools::strong(value)
}
),
URL = colDef(cell = function(value) {
# Render as a link
if (is.null(value) | is.na(value)) {
return("")
}
htmltools::a(href = value, target = "_blank", as.character(value))
}),
Phone = colDef(cell = function(value) {
# Render as a link
if (is.null(value) | is.na(value)) {
return("")
}
clearphone <- gsub("-", "", value)
clearphone <- gsub(" ", "", clearphone)
htmltools::a(
href = paste0("tel:", clearphone), target = "_blank",
as.character(value)
)
})
)
)
Session info
Details
#> ─ Session info ───────────────────────────────────────────────────────────────
#> setting value
#> version R version 4.4.2 (2024-10-31 ucrt)
#> os Windows Server 2022 x64 (build 20348)
#> system x86_64, mingw32
#> ui RTerm
#> language en
#> collate English_United States.utf8
#> ctype English_United States.utf8
#> tz UTC
#> date 2024-11-21
#> pandoc 3.1.11 @ C:/HOSTED~1/windows/pandoc/31F387~1.11/x64/PANDOC~1.11/ (via rmarkdown)
#>
#> ─ Packages ───────────────────────────────────────────────────────────────────
#> package * version date (UTC) lib source
#> arcgeocoder * 0.2.0 2024-11-21 [1] local
#> bslib 0.8.0 2024-07-29 [1] RSPM
#> cachem 1.1.0 2024-05-16 [1] RSPM
#> cli 3.6.3 2024-06-21 [1] RSPM
#> crosstalk * 1.2.1 2023-11-23 [1] RSPM
#> desc 1.4.3 2023-12-10 [1] RSPM
#> digest 0.6.37 2024-08-19 [1] RSPM
#> dplyr * 1.1.4 2023-11-17 [1] RSPM
#> evaluate 1.0.1 2024-10-10 [1] RSPM
#> fansi 1.0.6 2023-12-08 [1] RSPM
#> fastmap 1.2.0 2024-05-15 [1] RSPM
#> fs 1.6.5 2024-10-30 [1] RSPM
#> generics 0.1.3 2022-07-05 [1] RSPM
#> glue 1.8.0 2024-09-30 [1] RSPM
#> htmltools 0.5.8.1 2024-04-04 [1] RSPM
#> htmlwidgets 1.6.4 2023-12-06 [1] RSPM
#> httpuv 1.6.15 2024-03-26 [1] RSPM
#> jquerylib 0.1.4 2021-04-26 [1] RSPM
#> jsonlite 1.8.9 2024-09-20 [1] RSPM
#> knitr 1.49 2024-11-08 [1] RSPM
#> later 1.3.2 2023-12-06 [1] RSPM
#> leaflet * 2.2.2 2024-03-26 [1] RSPM
#> leaflet.providers 2.0.0 2023-10-17 [1] RSPM
#> lifecycle 1.0.4 2023-11-07 [1] RSPM
#> magrittr 2.0.3 2022-03-30 [1] RSPM
#> mime 0.12 2021-09-28 [1] RSPM
#> pillar 1.9.0 2023-03-22 [1] RSPM
#> pkgconfig 2.0.3 2019-09-22 [1] RSPM
#> pkgdown 2.1.1 2024-09-17 [1] any (@2.1.1)
#> promises 1.3.0 2024-04-05 [1] RSPM
#> purrr 1.0.2 2023-08-10 [1] RSPM
#> R.cache 0.16.0 2022-07-21 [1] RSPM
#> R.methodsS3 1.8.2 2022-06-13 [1] RSPM
#> R.oo 1.27.0 2024-11-01 [1] RSPM
#> R.utils 2.12.3 2023-11-18 [1] RSPM
#> R6 2.5.1 2021-08-19 [1] RSPM
#> ragg 1.3.3 2024-09-11 [1] RSPM
#> Rcpp 1.0.13-1 2024-11-02 [1] RSPM
#> reactable * 0.4.4 2023-03-12 [1] RSPM
#> reactR 0.6.1 2024-09-14 [1] RSPM
#> rlang 1.1.4 2024-06-04 [1] RSPM
#> rmarkdown 2.29 2024-11-04 [1] RSPM
#> sass 0.4.9 2024-03-15 [1] RSPM
#> sessioninfo * 1.2.2 2021-12-06 [1] any (@1.2.2)
#> shiny 1.9.1 2024-08-01 [1] RSPM
#> styler 1.10.3 2024-04-07 [1] RSPM
#> systemfonts 1.1.0 2024-05-15 [1] RSPM
#> textshaping 0.4.0 2024-05-24 [1] RSPM
#> tibble 3.2.1 2023-03-20 [1] RSPM
#> tidyselect 1.2.1 2024-03-11 [1] RSPM
#> utf8 1.2.4 2023-10-22 [1] RSPM
#> vctrs 0.6.5 2023-12-01 [1] RSPM
#> withr 3.0.2 2024-10-28 [1] RSPM
#> xfun 0.49 2024-10-31 [1] RSPM
#> xtable 1.8-4 2019-04-21 [1] RSPM
#> yaml 2.3.10 2024-07-26 [1] RSPM
#>
#> [1] D:/a/_temp/Library
#> [2] C:/R/site-library
#> [3] C:/R/library
#>
#> ──────────────────────────────────────────────────────────────────────────────